Are you preparing for a Spring Boot interview? Whether you’re a seasoned developer or new to the framework, being well-prepared can make all the difference. This post covers essential Spring Boot interview questions that can help you ace your interview. We will delve into core concepts, common configurations, and practical scenarios you might encounter. Brush up on these questions to demonstrate your expertise and confidence during your interview. Let’s get you ready to impress!
Table of Contents
ToggleTable of Contents
What is Sping Boot?
Imagine you’re building a Lego house. Normally, you’d need a ton of instruction manuals for all the different pieces (like windows, doors, etc.) and how to fit them together.
Spring Boot is like a super-instruction manual for building Java applications. It simplifies things by:
- Pre-sorting the Legos: Spring Boot comes with pre-built components for common tasks (like connecting to a database or creating a website). These are like having all the windows and doors already bagged and labeled.
- Automatic Instructions: Spring Boot can often figure out how to connect these components itself, saving you time writing a bunch of instructions. It’s like the manual practically writing itself!
- Easy Customization: Even though things are pre-built, you can still customize them if needed. Think of it like having extra Legos to add your own personal touch to the house.
Overall, Spring Boot makes building Java applications faster and easier, letting you focus on the unique features of your project instead of getting bogged down in setup.
How to Prepare for a Java Spring Boot Interview Questions?
Here’s a breakdown on how you can prepare for a Java Spring Boot Interview:
Solidify your Spring Boot Fundamentals:
- Core Concepts: Grasp core concepts like auto-configuration, dependency injection, Spring annotations (@SpringBootApplication, @Configuration, @Bean etc.), and Spring Boot starters.
- Building a Spring Boot App: Understand how to set up a project using Spring Initializr https://start.spring.io/, including common dependencies (Spring Web, Spring Data JPA etc.).
- Auto-Configuration: Be familiar with how Spring Boot automatically configures beans based on the included libraries.
Dive Deeper into Key Areas:
- Spring MVC: If building web applications, understand Spring MVC for handling requests and responses.
- Spring Data JPA: Explore data access with Spring Data JPA, including entity creation, repository usage, and JPA functionalities.
- Spring Security (Optional): For applications requiring authentication and authorization, understand basic Spring Security concepts.
Practice and Sharpen your Skills:
- Build Sample Applications: Create personal projects using Spring Boot to solidify your understanding and showcase your abilities in the interview.
- Online Resources: Utilize online resources like tutorials, practice questions, and mock interviews to test your knowledge under pressure.
- Spring Boot Documentation: Refer to the official Spring Boot documentation https://spring.io/guides/gs/spring-boot for in-depth explanations and examples.
Be Ready for Common Interview Questions:
- Spring Boot Advantages: Explain the benefits of using Spring Boot (reduced configuration, faster development etc.).
- Auto-Configuration: Discuss how Spring Boot auto-configures beans and how to customize it if needed.
- Starter Dependencies: Explain the purpose of Spring Boot starter dependencies and how to choose them for your project.
- Dependency Injection: Demonstrate your understanding of dependency injection and its role in Spring Boot applications.
- Building RESTful APIs (if applicable): If the role involves building APIs, be prepared to discuss RESTful APIs and Spring Boot’s approach to creating them.
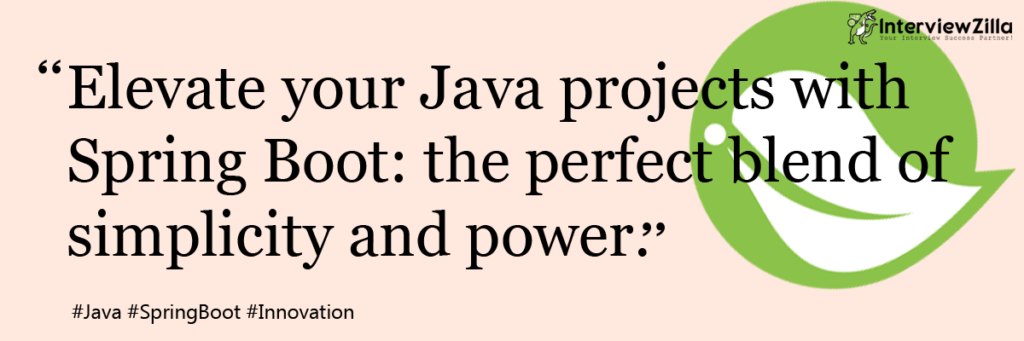
Top 40 Java Spring Boot Interview Questions and Answers
Q1. What is Spring Boot and why is it popular?
Ans: Spring Boot is a Java-based, convention-over-configuration framework widely recognized for simplifying Java application development. Its popularity stems from its ability to significantly reduce manual setup and boilerplate code, making it a preferred choice for developers. With an emphasis on defaults and simplicity, Spring Boot allows for the rapid creation of production-ready applications, addressing the complexities associated with traditional Spring configurations.
Q2. Explain the difference between Spring and Spring Boot?
Ans: Spring is a comprehensive Java framework offering modular support for various functionalities, while Spring Boot is an opinionated extension designed for streamlined development. The key difference lies in Spring Boot’s convention-over-configuration approach, minimizing the need for explicit setup. While Spring provides extensive flexibility, Spring Boot prioritizes simplicity and accelerates development by reducing configuration complexities.
Q3. How does Spring Boot simplify the development of Java applications?
Ans: Spring Boot simplifies Java development by providing convention-based configurations, sensible defaults, and an embedded server. This approach minimizes the need for extensive manual configurations, allowing developers to focus on writing application logic. The framework expedites development, making it easier to create production-ready applications with reduced setup efforts and a standardized project structure.
Q4. What is the purpose of the @SpringBootApplication annotation?
Ans: The @SpringBootApplication
annotation consolidates three essential annotations – @Configuration
, @EnableAutoConfiguration
, and @ComponentScan
. Placed on the main class, it serves as a unified entry point for the application, enabling auto-configuration, component scanning, and providing a streamlined starting point for development.
Q5. How does Spring Boot support auto-configuration?
Ans: Spring Boot supports auto-configuration by analyzing the classpath and automatically configuring the application based on the present dependencies. This intelligent configuration eliminates the need for extensive manual setup, allowing developers to focus on writing application code rather than intricate configurations.
Q6. What are Spring Boot Starters, and why are they used?
Ans: Spring Boot Starters are pre-configured sets of dependencies that simplify the inclusion of specific functionalities in an application. They streamline project setup by providing convenient dependency descriptors, promoting consistency across projects, and reducing the effort needed for configuring common features like web, data, or security.
Q7. Explain the significance of embedded servers in Spring Boot?
Ans: Embedded servers in Spring Boot eliminate the need for external server configurations. They allow the application to run as a self-contained unit, simplifying deployment and making it easier to develop, test, and deploy applications without relying on external server setups.
Q8. How does Spring Boot handle external configuration?
Ans: Spring Boot handles external configuration through property files, such as application.properties
or application.yml
. These files contain key-value pairs for configuring various settings, providing flexibility without modifying the source code. Externalized configuration is crucial for adapting applications to different environments without code changes.
Q9. What is the purpose of the application.properties file in Spring Boot?
Ans: The application.properties
file serves as a central configuration file in Spring Boot. It allows developers to specify key-value pairs to configure various aspects of the application, such as database connections, server ports, or external service URLs. This externalized configuration promotes maintainability and flexibility in different deployment scenarios.
Q10. How does Spring Boot support the development of microservices?
Ans: Spring Boot simplifies microservices development by providing features like embedded servers, easy configuration, and built-in support for RESTful services. It encourages the creation of loosely coupled, independently deployable microservices, aligning with modern microservices architecture principles.
Q11. Explain the role of Spring Boot Actuator in an application?
Ans: Spring Boot Actuator enhances the manageability of an application in production by providing features such as health checks, metrics, and monitoring. It exposes various endpoints that help monitor and manage the application’s health, performance, and behavior in a production environment.
Q12. What is the significance of the @RestController annotation in Spring Boot?
Ans: The @RestController
annotation in Spring Boot combines the @Controller
and @ResponseBody
annotations, making it specifically tailored for building RESTful web services. It simplifies the creation of REST endpoints by automatically serializing responses to JSON or XML, promoting efficient development of APIs.
Q13. How does Spring Boot handle database operations using Spring Data JPA?
Ans: Spring Boot simplifies database operations by seamlessly integrating with Spring Data JPA. It provides high-level abstractions, such as repositories and automatic CRUD operations, reducing the amount of boilerplate code needed for database access. This integration enhances productivity and maintains a cleaner codebase.
Q14. Explain the concept of Spring Boot Profiles?
Ans: Spring Boot Profiles allow developers to define different configurations for various environments, such as development, testing, or production. By activating specific profiles, the application adapts its behavior and settings according to the targeted environment, ensuring a seamless transition between different deployment scenarios.
Q15. How does Spring Boot support internationalization and localization?
Ans: Spring Boot supports internationalization and localization by providing properties files for different languages and locales. Developers can define messages, labels, and other text elements in multiple languages, making it easier to create applications that cater to a global audience with diverse language preferences.
Q16. What is the purpose of the @Autowired annotation in Spring Boot?
Ans: The @Autowired
annotation in Spring Boot facilitates automatic dependency injection. When applied to a field, constructor, or method, it signals to the Spring framework to automatically resolve and inject the required dependencies, reducing the need for manual bean wiring and promoting code readability.
Q17. How does Spring Boot support RESTful web services development?
Ans: Spring Boot streamlines RESTful web services development by providing annotations like @RestController
. These annotations simplify the creation of REST endpoints, making it easier for developers to build APIs that adhere to REST principles. Automatic serialization and deserialization further enhance the developer experience.
Q18. Explain the difference between @Component, @Service, and @Repository annotations?
Ans: In Spring Boot, @Component
is a generic stereotype for any Spring-managed component, @Service
is used for service components, and @Repository
is used for components that interact with databases. These annotations help categorize and autodiscover beans during component scanning, providing clarity and structure to the application.
Q19. What is the purpose of the @Value annotation in Spring Boot?
Ans: The @Value
annotation in Spring Boot is used for injecting values from properties files, environment variables, or other sources into beans. It simplifies the retrieval of configuration properties, allowing developers to access and use externalized configuration values within their components.
Q20. How does Spring Boot handle transaction management?
Ans: Spring Boot simplifies transaction management through its integration with Spring’s @Transactional
annotation. Developers can apply this annotation to methods, defining transactional boundaries and ensuring that a series of operations either succeed together or fail together, maintaining data consistency in a transactional context. This annotation promotes the use of declarative transactions, reducing boilerplate code related to transaction handling.
Q21. What is Spring Boot Initializr, and how is it used?
Ans: Spring Boot Initializr is a web-based tool that simplifies the process of bootstrapping a new Spring Boot project. Developers can use Initializr to generate a project structure with predefined settings, dependencies, and configurations based on their requirements. It provides a user-friendly interface for customizing project details and downloading the generated project as a ZIP file, expediting the setup process.
Q22. Explain the purpose of the @RequestMapping annotation in Spring Boot?
Ans: The @RequestMapping
annotation in Spring Boot is used to map HTTP requests to specific controller methods. It allows developers to define the URL patterns and HTTP methods that trigger the execution of a particular method. This annotation plays a crucial role in routing and handling incoming requests, serving as a bridge between the client and the corresponding controller method.
Q23. How does Spring Boot handle exception handling in RESTful services?
Ans: Spring Boot simplifies exception handling in RESTful services through the use of the @ControllerAdvice
annotation. This annotation allows developers to define global exception handling logic in a centralized manner, ensuring a consistent approach to handling exceptions across multiple controllers. By customizing exception handlers, developers can provide meaningful responses and maintain a standardized error-handling mechanism.
Q24. What is the significance of the CommandLineRunner interface in Spring Boot?
Ans: The CommandLineRunner
interface in Spring Boot is used to execute code after the application context has been fully loaded. It is often employed for tasks such as database initialization, data loading, or any post-processing logic that needs to run at the startup of the application. This interface provides a convenient hook for executing code as part of the application’s initialization process.
Q25. How does Spring Boot support WebSocket-based applications?
Ans: Spring Boot supports WebSocket-based applications by providing built-in support for WebSocket communication. Developers can use the @MessageMapping
annotation to handle WebSocket messages, and the integration with the SockJS library ensures compatibility with browsers that do not support WebSocket natively. This feature enables the development of real-time, bidirectional communication channels between clients and the server.
Q26. Explain the difference between @RequestParam and @PathVariable in Spring Boot?
Ans: In Spring Boot, @RequestParam
is used to extract values from query parameters in the URL, while @PathVariable
is used to extract values from the URI path. While @RequestParam
is typically used for non-path parameters, @PathVariable
is employed for variables embedded in the URL path. Understanding when to use each annotation is crucial for effectively handling client inputs.
Q27. What is the purpose of the SpringApplication class in Spring Boot?
Ans: The SpringApplication
class in Spring Boot provides a convenient way to bootstrap a Spring application. It allows developers to customize the application’s behavior, set properties, and configure settings programmatically before the application starts. This class plays a central role in initializing the Spring ApplicationContext and launching the application.
Q28. How does Spring Boot support asynchronous programming?
Ans: Spring Boot supports asynchronous programming through the @Async
annotation. When applied to a method, it indicates that the method should be executed asynchronously, allowing the application to perform other tasks while waiting for the asynchronous operation to complete. This feature enhances the responsiveness of the application and optimizes resource utilization.
Q29. Explain the use of the @CrossOrigin annotation in Spring Boot?
Ans: The @CrossOrigin
annotation in Spring Boot is used to enable Cross-Origin Resource Sharing (CORS) for specific controller methods. It allows web pages from one domain to make requests to a different domain, facilitating cross-origin communication. This annotation is essential for handling security considerations when dealing with cross-origin requests in a web application.
Q30. What is the purpose of the @ConditionalOnProperty annotation in Spring Boot?
Ans: The @ConditionalOnProperty
annotation in Spring Boot is used to conditionally enable or disable a component based on the presence or value of a configuration property. It allows developers to dynamically configure the behavior of the application by selectively activating or deactivating components based on specified conditions.
Q31. How does Spring Boot support caching mechanisms?
Ans: Spring Boot provides caching support through the @EnableCaching
annotation and integration with caching providers such as EhCache or Redis. Developers can annotate methods with @Cacheable
, @CacheEvict
, and @CachePut
to control caching behavior, improving application performance by reducing redundant computations or database queries.
Q32. Explain the difference between Spring Boot and Spring Cloud?
Ans: While Spring Boot focuses on simplifying the development of stand-alone, production-ready applications, Spring Cloud extends these capabilities to build distributed systems and microservices architectures. Spring Cloud provides tools and frameworks for building scalable, distributed applications, addressing challenges related to service discovery, configuration management, and distributed communication.
Q33. What is Spring Boot DevTools, and how does it enhance development?
Ans: Spring Boot DevTools is a set of tools aimed at enhancing the development experience. It provides features such as automatic application restarts, LiveReload support, and additional development-time features. DevTools aids developers in iterating quickly during the development phase, improving productivity and reducing the turnaround time for code changes.
Q34. How can you deploy a Spring Boot application?
Ans: Spring Boot applications can be deployed using various methods, including packaging as executable JAR or WAR files, deploying to standalone servers, or using containerization platforms like Docker. The choice depends on specific deployment requirements and infrastructure considerations, providing flexibility in deploying applications across different environments.
Q35. Explain the purpose of the @Async annotation in Spring Boot?
Ans: The @Async
annotation in Spring Boot is used to indicate that a method should be executed asynchronously. It allows developers to perform tasks concurrently, improving application responsiveness and resource utilization. This annotation is particularly beneficial for offloading time-consuming operations to separate threads, preventing blocking and enhancing overall system performance.
Q36. How does Spring Boot handle security in applications?
Ans: Spring Boot provides security features through the integration of Spring Security. Developers can secure their applications by configuring authentication, authorization, and other security-related settings using annotations, properties, or Java configuration. Spring Security simplifies the implementation of robust security measures, ensuring the protection of sensitive data and resources.
Q37. What is the role of the @RepositoryRestResource annotation in Spring Boot?
Ans: The @RepositoryRestResource
annotation in Spring Boot is used to expose Spring Data repositories as RESTful endpoints. It automatically generates RESTful CRUD operations for entities, simplifying the creation of RESTful APIs for data access. This annotation streamlines the process of exposing and accessing data through HTTP endpoints.
Q38. How can you customize error pages in a Spring Boot application?
Ans: Error pages in Spring Boot can be customized by creating error templates in the src/main/resources/templates/error
directory. Additionally, developers can customize error handling by creating a class annotated with @ControllerAdvice
to handle specific error scenarios. This customization allows developers to provide personalized error messages and responses, improving the user experience during error conditions.
Q39. Explain the purpose of the @Configuration annotation in Spring Boot?
Ans: The @Configuration
annotation in Spring Boot indicates that a class contains bean definitions and configuration settings. It plays a crucial role in Java-based configuration, allowing developers to define beans, configure components, and customize the application context. This annotation is integral to the modular and organized configuration of Spring Boot applications.
Q40. How does Spring Boot handle the creation of executable JARs or WARs?
Ans: Spring Boot simplifies the creation of executable JARs or WARs by providing the spring-boot-maven-plugin
or spring-boot-gradle-plugin
. These plugins package the application with its dependencies, including an embedded server, into a single executable file. This approach eliminates the need for an external server, making deployment straightforward and allowing for easy execution of the application with a simple command.
Click here for more Java related topics.
To know more about Java please visit Java official site.
Like This? Share with your Friends and Colleagues
- Click to share on LinkedIn (Opens in new window) LinkedIn
- Click to share on Facebook (Opens in new window) Facebook
- Click to share on X (Opens in new window) X
- Click to email a link to a friend (Opens in new window) Email
- Click to share on Telegram (Opens in new window) Telegram
- Click to print (Opens in new window) Print
- Click to share on WhatsApp (Opens in new window) WhatsApp